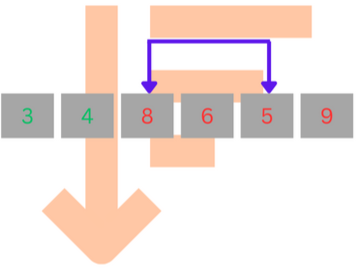
Sorting and searching are two fundamental operations in computer science.
Sorting refers to the process of arranging a collection of items in a specific order, typically in ascending or descending order based on a certain criteria.
Searching, on the other hand, is the process of finding a specific item or value within a collection of items.
Sorting and searching operations goes hand in hand this is partly because it is far easier to locate a specific element when the dataset is sorted than when it is unsorted. Thus sorting greatly improves search efficiency and hence data retrieval.
Both sorting and searching algorithms are used widely in all sort of applications. Understanding them is a fundamental part of programming and computer science in general.
In this article you will get a glimpse on the various sorting and searching algorithms without immersing into intricate implementation details.
Sorting algorithms
Sorting algorithms are used to arrange data in a specific order, usually from smallest to largest or vice versa. This makes it easier to search for specific items as well as to perform other operations on the data.
Sorting algorithms can be broadly classified into two categories:
- comparison-based sorting
- non-comparison-based sorting.
Comparison-based sorting algorithms compare elements in a specific order and make decisions based on the result of the comparison. Examples of comparison-based sorting algorithms include
- bubble sort.
- selection sort
- insertion sort.
- merge sort
- quick sort.
Non-comparison-based sorting algorithms do not rely on comparisons between elements. Instead, they use the existing order of the elements to sort them efficiently. The include:
- Counting sort
- Bucket sort.
- Radix sort.
Differences between comparison and non-comparison-based sorting algorithms
comparison based | non-comparison based |
---|---|
Compares elements to determine their relative ordering | Do not depend on comparisons |
Can work on any type of data, as long as comparisons can be made between elements | Are restricted to certain types of data. |
Applied in general sorting problems. | Are applied in very specific sorting problems. |
Can have a worst-case running time of O(n2) |
Generally have better worst-case running times. |
Searching Algorithms
Searching algorithms are used to find a given item in a dataset. They can be categorized into two:
- linear search
- binary search.
Linear search involves sequentially checking each element in a collection until the desired item is found or the end of the collection is reached. Binary search, on the other hand, is more efficient as it divides the search space in half with each iteration, reducing the number of elements to search and thus improving the overall performance.