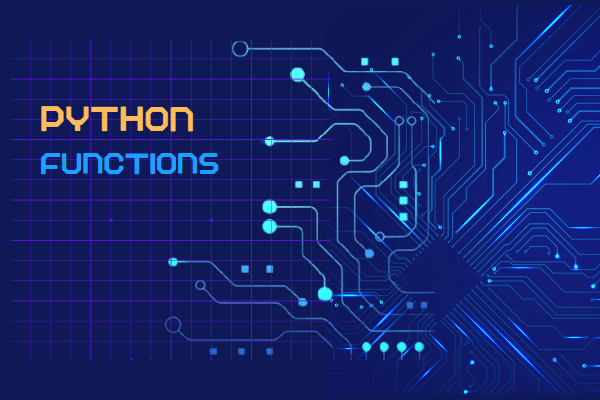
A function is a group of statements that are wrapped together to perform a specific task. Unless you are absolutely new to Python and programming in general, you have most likely already interacted with functions . Some common functions in Python include:
- The
print()
function - Used to output values to the console - The
input()
function - used to get user inputs. - The
len()
function - used to get the length of an iterable object such as lists, strings, tuples, etc. - The
range()
function - used to get values in a given range
The above functions, and many others, comes pre-built in the Python interpreter meaning that we just call them without any necessary setups, importations or installations. For example to use the print()
function, you simply call it with the necessary arguments, as shown below:
print('Hello, World!')
We can also create our own functions which we can use to perform some repeating tasks, or to break a task into subtasks in our program. These type of functions are known as user-defined functions.
All Functions, whether in-built or user-defined, typically go through three major stages i.e declaration, implementation and usage/calling. In in-built functions, declaration and implementation are already done for us, therefore, we just use/call the functions. However, in user-defined functions we have to declare and implement the functions ourselves before using/ calling them. You can check more on Function declaration and implementation here.
Why use Functions?
Functions provide numerous benefits that improve code quality, the following are some of of the reasons why we need to use functions in our programs:
-
Code Reusability.
Python functions are the most basic factoring tool in the language, they allow us to reduce code repetition in our programs. A function, once defined, can be called whenever needed thus making the statements inside its block highly reusable. Without functions, we would need to repeat the same set of instructions each time we want to perform a task, leading to longer and more complex programs.
-
Code Modularity
Functions helps to break a task into logical and manageable pieces, which eases the development process. For example in an image processing program, we might break the program into several functions, such as one to resize images, another to crop images, another to apply filters etc. This makes the program to be easily manageable.
-
Easy Maintenance
When we need to make changes to code that occurs multiple times in a program, it can be time-consuming and error-prone to update each occurrence of the code manually. However, if the code is found within a function, we only need to update the function once, and the changes will take effect wherever the function is called in the program. This makes it easier to maintain and update our programs over time.
-
Code Readability
Functions help us group statements together under a common and intuitive name. For example one will easily tell what a function called resize_image is intended to accomplish, than would for bare statements that are intended to perform the same task. Functions, therefore, help improve readability by making code more organized and easier to understand. When we use descriptive names for functions, it becomes easier for other developers to quickly understand what each function does without having to read through the entire code. Each function can also have its own documentation string that describes its purpose in details.
-
Code abstraction
Functions can be used to abstract complex processes in a program. This can help to keep the program friendly to future users and maintainers by exposing only the functionality of the a function but not its implementation. For example, most of Python users barely knows how most builtin functions such as
print()
are implemented despite using them regularly in their programs.Functions can also be tailored to function differently depending on some given circumstances, such as the operating system the user is on.
-
Testing and Debugging
When errors occur in a Program, Python includes, in the Traceback, the functions which were called before the error , this can simplify the debugging process because one will know which functions to look first before trying other debugging options. It is also easier to test statements inside a function than it is for bare statements.
The three main types of functions in Python are the built-in functions, the user-defined functions and the lambda functions. We will look more on each of these functions in the following articles.